Hooks
With the JetBackup Hooks System, you can trigger custom scripts when JetBackup executes an task on specific hook points. For example, you could use hooks to notify you through your preferred application when a backup job starts, completes or fails.
Create New Hook
To create a new hook, click on "+ Create New Hook".
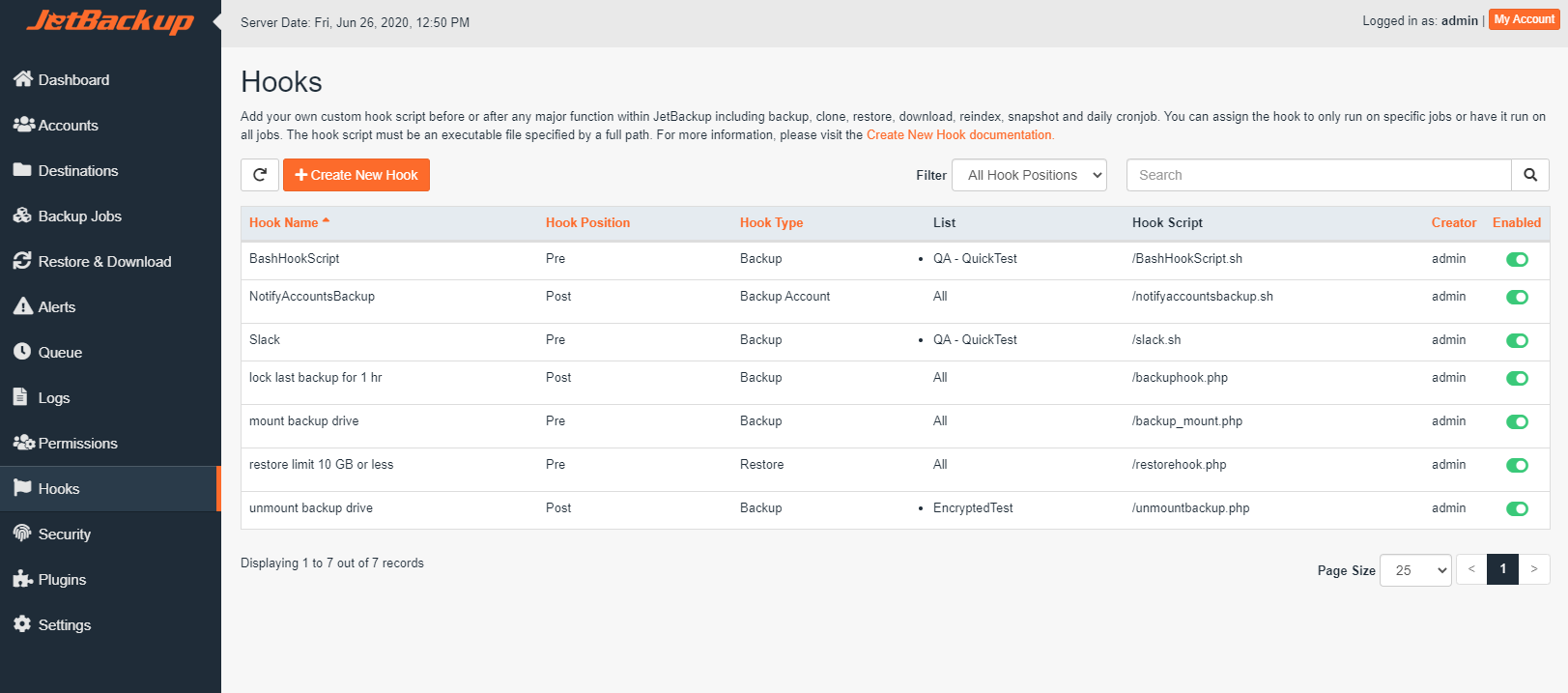
Example configuration page for hooks:
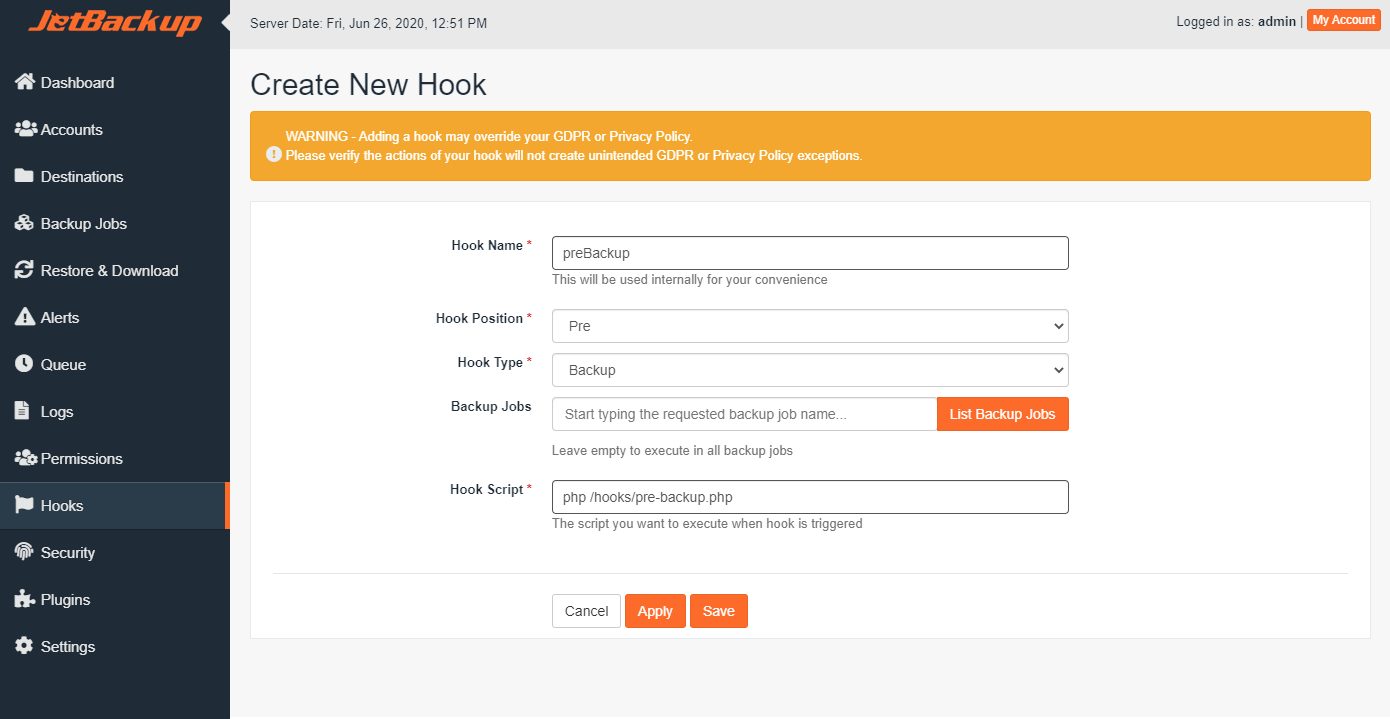
Here's a sample script that goes through each parameter passed from the hook and prints
the key(parameter name) and value of each parameter to a "testFile".
#!/usr/bin/php
// PHP sample for JetBackup hook
// All Hook Args are stored in $argv
<?php
for ($i = 1; $i < sizeof($argv); $i++) {
$data = "Arg " . $i . ": " . $argv[$i] . "\r\n"; //Format Data
file_put_contents("/root/testFile", $data, FILE_APPEND); //Append each arg to file
}
?>
#!/bin/bash
# Hook Args are passed into $@
echo Printing Hook Parameters: >> /root/testFile
argArray=( "$@" ) # Storing args in new array for printing
for i in "${!argArray[@]}"; do
echo "Arg $i: ${argArray[$i]}" >> /root/testFile
done
#!/usr/bin/python
#Python sample for JetBackup 5 Hook
import sys
f = open("/root/PythonTestFile", 'w')
f.write("Printing Hook Parameters:\n")
for i in range(1,len(sys.argv)):
f.write("Arg "+str(i)+": "+sys.argv[i]+"\n")
f.close()
Hook Name
This will be used internally your convenience.
Hook Position
Choose to run your script Pre/Post selected task.
Hook Type
The hook type defines which task the hook should be executed.
Each hook type receives a different set of arguments.
Your script can fetch this data and use it to its own needs.
These are the available action type and the arguments that are passed:
Backup Hook
Pre Backup Hook Args
Pre Backup
Argument Index |
Value |
Possible Values |
$1 |
Backup Job ID |
N/A |
$2 |
Backup Job Name |
N/A |
$3 |
Backup Job Type |
- 1 - Accounts
- 2 - Directories
- 3 - JB Config
|
$4 |
Backup Job Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$5 |
Backup Job Structure |
- 1 - Incremental
- 2 - Archived
- 4 - Compressed
|
$6 |
Backup Job Destinations: All Destination IDs are seperated by a comma ,. |
N/A |
$7 |
Backup Start Date (EPOCH Time) |
N/A |
$8 |
Backup Log ID |
N/A |
Post Backup Hook Args
Post Backup
Argument Index |
Value |
Example |
$1 |
Backup Job ID |
N/A |
$2 |
Backup Job Name |
N/A |
$3 |
Backup Job Type |
- 1 - Accounts
- 2 - Directories
- 3 - JB Config
|
$4 |
Backup Job Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$5 |
Backup Job Structure |
- 1 - Incremental
- 2 - Archived
- 4 - Compressed
|
$6 |
Backup Job Destinations: All Destination IDs are seperated by a comma ,. |
N/A |
$7 |
Backup Start Date (EPOCH Time UTC) |
N/A |
$8 |
Backup End Date (EPOCH Time UTC) |
N/A |
$9 |
Backup Status (Queue Object Status) |
- 1 - Pending
- 2 - Processing
- 100 - Completed
- 101 - Partially Completed
- 102 - Failed
- 103 - Aborted
- 104 - Never Finished
|
$10 |
Backup Log ID |
N/A |
Backup Account Hook
Pre Backup Account Hook Args
Pre Backup Account
Argument Index |
Value |
Possible Values |
$1 |
Backup Job ID |
N/A |
$2 |
Account ID |
N/A |
$3 |
Account User Name |
N/A |
$4 |
Backup Account Log ID |
N/A |
Post Backup Account Hook Args
Post Backup Account
Argument Index |
Value |
Example |
$1 |
Backup Job ID |
N/A |
$2 |
Account ID |
N/A |
$3 |
Account User Name |
N/A |
$4 |
Backup Account Status |
- 1 - Completed
- 2 - Partially Completed
- 3 - Failed
- 4 - Aborted
|
$5 |
Backup Account Log ID |
N/A |
$6 |
Queue Object Status |
- 100 - Completed
- 101 - Partially Completed
- 102 - Failed
- 103 - Aborted
|
Clone Hook
Pre Clone Hook Args
Pre Clone
Argument Index |
Value |
Possible Values |
$1 |
Clone Job ID |
N/A |
$2 |
Clone Job Name |
N/A |
$3 |
Clone Job Type |
|
$4 |
Clone Job Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$5 |
Clone Job Destinations: All Destination IDs are seperated by a comma ,. |
N/A |
$6 |
Clone Start Date (EPOCH Time) |
N/A |
$7 |
Clone Log ID |
N/A |
Post Clone Hook Args
Post Clone
Argument Index |
Value |
Example |
$1 |
Clone Job ID |
N/A |
$2 |
Clone Job Name |
N/A |
$3 |
Clone Job Type |
|
$4 |
Clone Job Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$5 |
Clone Job Destinations: All Destination IDs are seperated by a comma ,. |
N/A |
$6 |
Clone Start Date (EPOCH Time UTC) |
N/A |
$7 |
Clone End Date (EPOCH Time UTC) |
N/A |
$8 |
Clone Status (Queue Object Status) |
- 1 - Pending
- 2 - Processing
- 100 - Completed
- 101 - Partially Completed
- 102 - Failed
- 103 - Aborted
- 104 - Never Finished
|
$9 |
Clone Log ID |
N/A |
Clone Account Hook
Pre Clone Account Hook Args
Pre Clone Account
Argument Index |
Value |
Possible Values |
$1 |
Clone Job ID |
N/A |
$2 |
Account ID |
N/A |
$3 |
Account User Name |
N/A |
$4 |
Clone Account Log ID |
N/A |
Post Clone Account Hook Args
Post Clone Account
Argument Index |
Value |
Example |
$1 |
Clone Job ID |
N/A |
$2 |
Account ID |
N/A |
$3 |
Account User Name |
N/A |
$4 |
Clone Account Status |
N/A |
$4 |
Clone Account Log ID |
N/A |
Restore Hook
Pre Restore Hook Args
Argument Index |
Value |
Possible Values |
$1 |
Backup Type |
- 1 - Accounts
- 2 - Directories
- 3 - JB Config
|
$2 |
Backup Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$3 |
Account ID |
N/A |
$4 |
Account User Name |
N/A |
$5 |
Backup Items: All items are separated by a comma ,. |
N/A |
$6 |
Restore Start Date (Epoch Time UTC) |
N/A |
$7 |
Restore Log ID |
N/A |
Post Restore Hook Args
Argument Index |
Value |
Example |
$1 |
Backup Type |
- 1 - Accounts
- 2 - Directories
- 3 - JB Config
|
$2 |
Backup Contains: Bitwise Value - Full Account: 511 = 1 + 2 + 4 + 8 + 16 + 32 + 64 + 128 + 256 |
- 1 - Account Panel Config
- 2 - Account Home Directory
- 4 - Account Databases
- 8 - Account Email
- 16 - Cron Jobs
- 32 - Accounts Domain
- 64 - Account Certificates
- 128 - Database Users
- 256 - FTP Accounts
- 511 - Full Account
|
$3 |
Account ID |
The Account ID only exists for Account Restore Type. |
$4 |
Account Name |
Account User Name only exists for Account Restore Type. |
$5 |
Backup Items: All items are separated by a comma ,. |
N/A |
$6 |
Restore Start Date (Epoch Time UTC) |
N/A |
$7 |
Restore End Date (Epoch Time UTC) |
N/A |
$8 |
Restore Status (Queue Object Status) |
- 1 - Pending
- 2 - Processing
- 100 - Completed
- 101 - Partially Completed
- 102 - Failed
- 103 - Aborted
- 104 - Never Finished
|
$9 |
Restore Log ID |
N/A |
Download Hook
Pre Download Hook Args
Argument Index |
Value |
Possible Values |
$1 |
Account ID |
Account ID only exists for Account Backup Download. |
$2 |
Download Log ID |
N/A |
Post Download Hook Args
Argument Index |
Value |
Example |
$1 |
Account ID |
Account ID only exists for Account Backup Download. |
$2 |
Download Log ID |
N/A |
Hook Script
The hook script must be an executable file specified by full path.
For example:
If it's not an executable script, such as regular PHP file you should specify the executable to run your script.
For example:
/usr/bin/php /hooks/pre-backup.php
This executable should exit with success (0) if it executed successfully,
Otherwise, if it fails, it should exit with an error code - other than 0.
Hook Settings
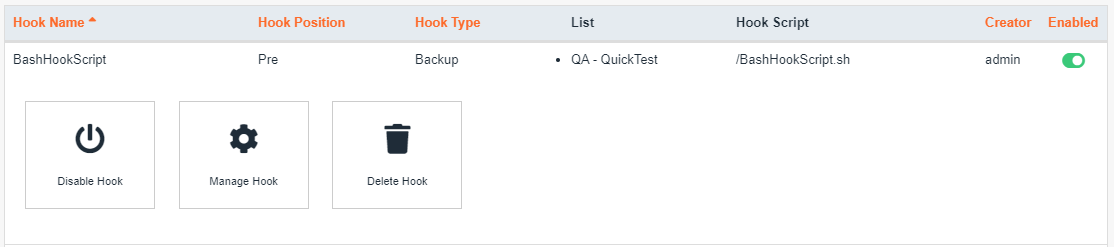
Enable/Disable Hook
Click on Disable/Enable Hook to Disable/Enable your Hook.
Manage Hook
Click on Manage Hook to modify Hook settings.
Delete Hook
Click on Delete Hook to Delete your Hook permanently.